Introduction
Metadata is information in an image file that includes details like the date, time, camera settings, and location. Accessing and changing this data is key for tasks such as organizing photos or analyzing images. This article will show you how to get an image’s metadata in Python, save an image as metadata, and add metadata to a JPG file.
Understanding Image Metadata
Before diving into the practical steps, it’s essential to understand what image metadata is. Metadata is divided into several categories:
- Exif (Exchangeable Image File Format): Contains information about the camera settings and conditions under which the image was taken.
- IPTC (International Press Telecommunications Council): Used for photojournalism and news media, containing information like captions, keywords, and authorship.
- XMP (Extensible Metadata Platform): A more modern format that can embed metadata into different types of media files.
How to Get Metadata of Image in Python
To extract metadata from an image in Python, we can use libraries such as PIL (Pillow) and exifread. Here’s a step-by-step guide:
Using PIL (Pillow)
Pillow is an image processing library in Python that allows you to manipulate and analyze images easily.
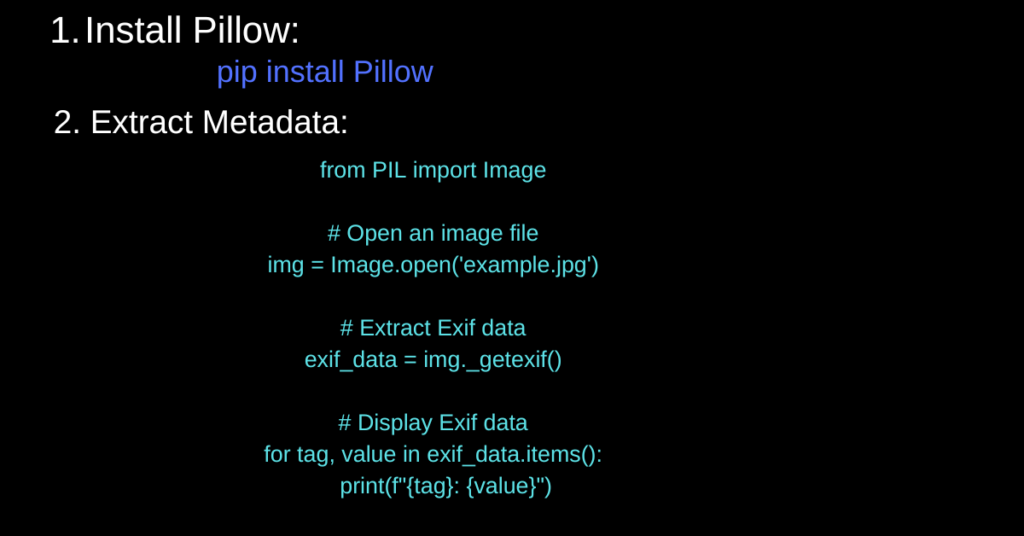
1. Install Pillow:
pip install Pillow
Extract Metadata:
from PIL import Image
# Open an image file
img = Image.open(‘example.jpg’)
# Extract Exif data
exif_data = img._getexif()
# Display Exif data
for tag, value in exif_data.items():
print(f”{tag}: {value}”)
Using exifread
Exifread is another useful library specifically designed for extracting Exif metadata.
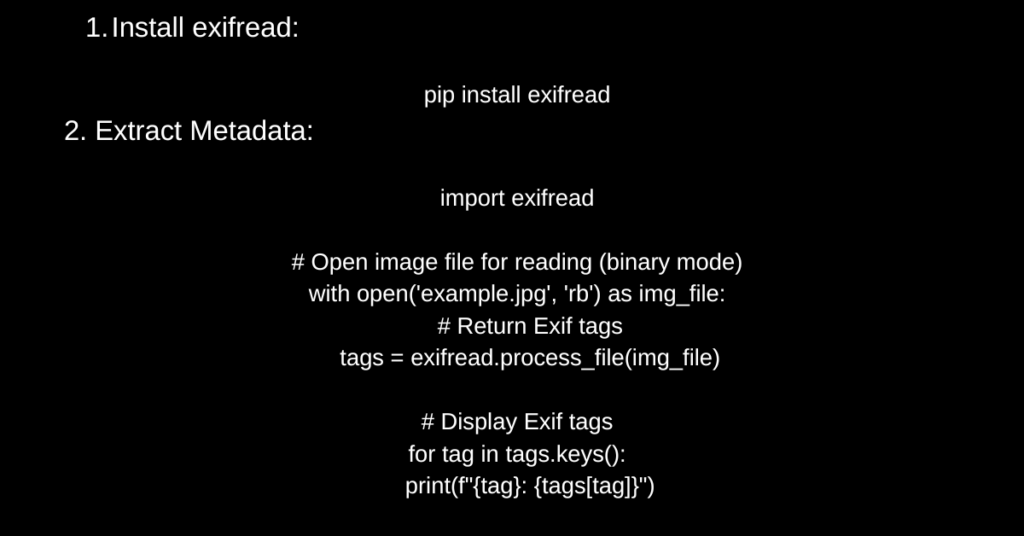
2. Install exifread:
pip install exifread
Extract Metadata:
import exifread
# Open image file for reading (binary mode)
with open(‘example.jpg’, ‘rb’) as img_file:
# Return Exif tags
tags = exifread.process_file(img_file)
# Display Exif tags
for tag in tags.keys():
print(f”{tag}: {tags[tag]}”)
How Do I Save an Image as Metadata?
Saving metadata involves embedding additional information within the image file itself. Here’s how you can save metadata using the piexif library:
Using piexif
Piexif is a library that simplifies the process of modifying Exif metadata in images.
3. Install piexif:
pip install piexif
Save Metadata:
import piexif
from PIL import Image
# Load image
img = Image.open(‘example.jpg’)
# Define Exif data
exif_dict = piexif.load(img.info[‘exif’])
# Modify Exif data (example: changing the Artist tag)
exif_dict[‘0th’][piexif.ImageIFD.Artist] = “Your Name”
# Insert Exif data back to image
exif_bytes = piexif.dump(exif_dict)
img.save(‘example_with_metadata.jpg’, exif=exif_bytes)
How Do You Add Metadata to a JPG?
Adding metadata to a JPG involves creating or modifying the metadata fields and then embedding them into the image. Here’s how you can add metadata using Pillow and piexif:
Using Pillow and piexif
Install Pillow and piexif:
pip install Pillow piexif
Add Metadata:
import piexif
from PIL import Image
# Open an image file
img = Image.open(‘example.jpg’)
# Create new Exif data
exif_dict = {
‘0th’: {
piexif.ImageIFD.Artist: “Your Name”,
piexif.ImageIFD.Make: “Your Camera Make”,
piexif.ImageIFD.Model: “Your Camera Model”
},
‘Exif’: {
piexif.ExifIFD.DateTimeOriginal: “2024:07:29 10:00:00”,
}
}
# Convert Exif data to bytes
exif_bytes = piexif.dump(exif_dict)
# Save the image with new Exif data
img.save(‘example_with_new_metadata.jpg’, exif=exif_bytes)
Using exiftool
ExifTool is a powerful command-line application for reading, writing, and editing metadata. Though it is not a Python library, it can be called from within a Python script using the subprocess module.
Install ExifTool:
# For Windows
choco install exiftool
# For macOS
brew install exiftool
Add Metadata:
import subprocess
# Command to add metadata
command = [
‘exiftool’,
‘-Artist=Your Name’,
‘-Make=Your Camera Make’,
‘-Model=Your Camera Model’,
‘-DateTimeOriginal=2024:07:29 10:00:00’,
‘-overwrite_original’,
‘example.jpg’
]
# Execute command
subprocess.run(command)
Conclusion
Extracting, saving, and adding metadata to images in Python is a valuable skill. It enhances the management of your image collections. Libraries like Pillow, exifread, and piexif, along with ExifTool, make this easy. Whether organizing photos, analyzing images, or developing software, understanding image metadata is crucial. This article guides you in efficiently handling image metadata, thus improving your workflows.
FAQs on How to Get Metadata of Image in Python
1. What is image metadata?
Image metadata is information embedded within an image file that provides details about the image. This can include data such as the date and time the image was taken, camera settings, location, author, and more.
2. Why is image metadata important?
Image metadata is important because it helps in organizing, searching, and managing image collections. It also provides context and additional information about the image, which can be useful for photographers, researchers, and developers.
3. How can I get metadata from an image using Python?
You can get metadata from an image in Python using libraries such as Pillow and exifread. These libraries allow you to read and extract Exif metadata embedded in image files.
4. What is Exif metadata?
Exif (Exchangeable Image File Format) metadata contains information about the camera settings and conditions under which the image was taken, such as shutter speed, aperture, ISO, focal length, and more.
5. How do I install the necessary libraries to work with image metadata in Python?
You can install the required libraries using pip. For example:
- To install Pillow: pip install Pillow
- To install exifread: pip install exifread
- To install piexif: pip install piexif
6. How do I extract Exif metadata using Pillow?
Here’s a basic example of how to extract Exif metadata using Pillow:
from PIL import Image
# Open an image file
img = Image.open(‘example.jpg’)
# Extract Exif data
exif_data = img._getexif()
# Display Exif data
for tag, value in exif_data.items():
print(f”{tag}: {value}”)
7. How do I save an image with metadata using Python?
You can save an image with metadata using the piexif library. Here is a simple example:
import piexif
from PIL import Image
# Load image
img = Image.open(‘example.jpg’)
# Define Exif data
exif_dict = piexif.load(img.info[‘exif’])
# Modify Exif data (example: changing the Artist tag)
exif_dict[‘0th’][piexif.ImageIFD.Artist] = “Your Name”
# Insert Exif data back to image
exif_bytes = piexif.dump(exif_dict)
img.save(‘example_with_metadata.jpg’, exif=exif_bytes)
8. How can I add metadata to a JPG file in Python?
To add metadata to a JPG file, you can use the piexif library. Here is how you can do it:
import piexif
from PIL import Image
# Open an image file
img = Image.open(‘example.jpg’)
# Create new Exif data
exif_dict = {
‘0th’: {
piexif.ImageIFD.Artist: “Your Name”,
piexif.ImageIFD.Make: “Your Camera Make”,
piexif.ImageIFD.Model: “Your Camera Model”
},
‘Exif’: {
piexif.ExifIFD.DateTimeOriginal: “2024:07:29 10:00:00”,
}
}
# Convert Exif data to bytes
exif_bytes = piexif.dump(exif_dict)
# Save the image with new Exif data
img.save(‘example_with_new_metadata.jpg’, exif=exif_bytes)
9. Can I use command-line tools to add metadata to images?
Yes, you can use command-line tools like ExifTool to add metadata to images. ExifTool is a powerful application that can be called from within a Python script using the subprocess module.
10. How do I install ExifTool?
You can install ExifTool using package managers. For example:
- On Windows: choco install exiftool
- On macOS: brew install exiftool
11. How do I add metadata to an image using ExifTool in Python?
You can use the subprocess module to call ExifTool from within a Python script. Here’s an example:
import subprocess
# Command to add metadata
command = [
‘exiftool’,
‘-Artist=Your Name’,
‘-Make=Your Camera Make’,
‘-Model=Your Camera Model’,
‘-DateTimeOriginal=2024:07:29 10:00:00’,
‘-overwrite_original’,
‘example.jpg’
]
# Execute command
subprocess.run(command)
12. Are there any limitations to the types of metadata I can add or modify?
While you can add or modify most types of metadata, the specific metadata fields available may vary depending on the format and capabilities of the image file and the tools you are using. Some metadata fields may also have constraints based on the image format standards.
13. Can I remove metadata from an image?
Yes, you can remove metadata from an image using libraries like piexif or tools like ExifTool. For example, using ExifTool, you can remove all metadata with the following command:
import subprocess
# Command to remove all metadata
command = [‘exiftool’, ‘-all=’, ‘example.jpg’, ‘-overwrite_original’]
# Execute command
subprocess.run(command)
14. How can I verify the metadata added to an image?
You can verify the metadata added to an image by extracting and reviewing the metadata using the same libraries or tools you used to add the metadata. For example, you can use Pillow, exifread, or ExifTool to read and display the metadata fields.